mirror of
https://github.com/WinampDesktop/winamp.git
synced 2025-05-18 20:15:42 -04:00
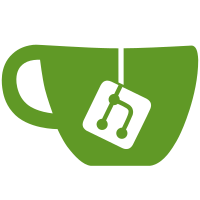
Also provides batch mode and automatic fullscreen switching. -help: Displays this information and exits. -version: Displays version information and exits. -batch: Enables batch mode (exits after powering off) -fastboot: Force fast boot for provided filename -slowboot: Force slow boot for provided filename -resume: Load resume save state. If a boot filename is provided, that game's resume state will be loaded, otherwise the most recent resume save state will be loaded. -state <index>: Loads specified save state by index. If a boot filename is provided, a per-game state will be loaded, otherwise a global state will be loaded. -statefile <filename>: Loads state from the specified filename. No boot filename is required with this option. -fullscreen: Enters fullscreen mode immediately after starting. -nofullscreen: Prevents fullscreen mode from triggering if enabled. -portable: Forces "portable mode", data in same directory. --: Signals that no more arguments will follow and the remaining parameters make up the filename. Use when the filename contains spaces or starts with a dash.
67 lines
1.8 KiB
C++
67 lines
1.8 KiB
C++
#include "common/assert.h"
|
|
#include "common/log.h"
|
|
#include "core/system.h"
|
|
#include "frontend-common/sdl_initializer.h"
|
|
#include "sdl_host_interface.h"
|
|
#include <SDL.h>
|
|
#include <cstdio>
|
|
#include <cstdlib>
|
|
|
|
#undef main
|
|
int main(int argc, char* argv[])
|
|
{
|
|
// set log flags
|
|
#ifndef _DEBUG
|
|
const LOGLEVEL level = LOGLEVEL_INFO;
|
|
// const LOGLEVEL level = LOGLEVEL_DEV;
|
|
// const LOGLEVEL level = LOGLEVEL_PROFILE;
|
|
Log::SetConsoleOutputParams(true, nullptr, level);
|
|
Log::SetFilterLevel(level);
|
|
#else
|
|
Log::SetConsoleOutputParams(true, nullptr, LOGLEVEL_DEBUG);
|
|
Log::SetConsoleOutputParams(true, "Pad DigitalController MemoryCard SPU", LOGLEVEL_DEBUG);
|
|
// Log::SetConsoleOutputParams(true, "GPU GPU_HW_OpenGL SPU Pad DigitalController", LOGLEVEL_DEBUG);
|
|
// Log::SetConsoleOutputParams(true, "GPU GPU_HW_OpenGL Pad DigitalController MemoryCard InterruptController SPU
|
|
// MDEC", LOGLEVEL_DEBUG); g_pLog->SetFilterLevel(LOGLEVEL_TRACE);
|
|
Log::SetFilterLevel(LOGLEVEL_DEBUG);
|
|
// Log::SetFilterLevel(LOGLEVEL_DEV);
|
|
#endif
|
|
|
|
FrontendCommon::EnsureSDLInitialized();
|
|
|
|
std::unique_ptr<SDLHostInterface> host_interface = SDLHostInterface::Create();
|
|
std::unique_ptr<SystemBootParameters> boot_params;
|
|
if (!host_interface->ParseCommandLineParameters(argc, argv, &boot_params))
|
|
{
|
|
SDL_Quit();
|
|
return EXIT_FAILURE;
|
|
}
|
|
|
|
if (!host_interface->Initialize())
|
|
{
|
|
host_interface->Shutdown();
|
|
SDL_Quit();
|
|
return EXIT_FAILURE;
|
|
}
|
|
|
|
if (boot_params)
|
|
{
|
|
if (!host_interface->BootSystem(*boot_params) && host_interface->InBatchMode())
|
|
{
|
|
host_interface->Shutdown();
|
|
host_interface.reset();
|
|
SDL_Quit();
|
|
return EXIT_FAILURE;
|
|
}
|
|
|
|
boot_params.reset();
|
|
}
|
|
|
|
host_interface->Run();
|
|
host_interface->Shutdown();
|
|
host_interface.reset();
|
|
|
|
SDL_Quit();
|
|
return EXIT_SUCCESS;
|
|
}
|